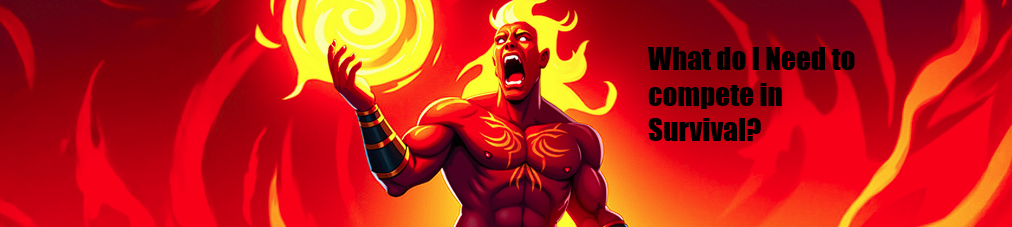
I wanted to see what Summoners I had left in survival mode and also how many monsters I had in each splinter too so I could decide if I wanted to pick up some additional summoners but I could not see an easy way to find that info in the Splinterlands filters. Yes you can see all the cards you have enabled, all the cards that are depleted but not all the enabled cards remaining..
I'm no programmer but I like data, so I thought a few questions to chatGPT and i would have an answer so i eventually got it to produce the below code.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Splinterlands Survival Mode Checker</title>
<script src="https://cdn.jsdelivr.net/npm/chart.js"></script>
<style>
table {
width: 100%;
border-collapse: collapse;
}
th, td {
border: 1px solid black;
padding: 8px;
text-align: left;
}
</style>
</head>
<body>
<h1>Splinterlands Survival Mode Checker</h1>
<label for="username">Enter Username:</label>
<input type="text" id="username" placeholder="Enter Splinterlands Username">
<button onclick="fetchSurvivalStatus()">Check Survival Mode</button>
<h2>Summoners</h2>
<table id="summonerTable">
<thead>
<tr>
<th>Card Name</th>
<th>Set</th>
<th>Splinter</th>
<th>Level</th>
</tr>
</thead>
<tbody></tbody>
</table>
<h2>Card Splinter Count</h2>
<table id="colorCountTable">
<thead>
<tr>
<th>Splinter</th>
<th>Count</th>
</tr>
</thead>
<tbody></tbody>
</table>
<h2>Monsters</h2>
<table id="monsterTable">
<thead>
<tr>
<th>Card Name</th>
<th>Set</th>
<th>Splinter</th>
<th>Level</th>
</tr>
</thead>
<tbody></tbody>
</table>
<script>
const splinterMapping = {
"red": "Fire",
"blue": "Water",
"green": "Earth",
"white": "Life",
"black": "Death",
"gold": "Dragon",
"gray": "Neutral"
};
const editionMapping = {
0: "Alpha",
1: "Beta",
2: "Promo",
3: "Reward",
4: "Untamed",
5: "Dice",
6: "Gladius",
7: "Chaos Legion",
8: "Riftwatchers",
9: "Rebellion"
};
async function fetchSurvivalStatus() {
const username = document.getElementById('username').value.trim();
if (!username) {
alert("Please enter a username.");
return;
}
const collectionUrl = `https://api.splinterlands.io/cards/collection/${username}`;
const detailsUrl = "https://api.splinterlands.io/cards/get_details";
try {
const [collectionResponse, detailsResponse] = await Promise.all([
fetch(collectionUrl),
fetch(detailsUrl)
]);
const collectionData = await collectionResponse.json();
const cardDetails = await detailsResponse.json();
if (!collectionData.cards) {
alert("No cards found for this user.");
return;
}
const cardDetailsMap = {};
const splinterCount = {};
cardDetails.forEach(detail => {
const color = detail.color ? detail.color.toLowerCase() : "gray";
const splinter = splinterMapping[color] || "Unknown";
cardDetailsMap[detail.id] = {
name: detail.name,
type: detail.type,
splinter: splinter
};
if (!splinterCount[splinter]) {
splinterCount[splinter] = 0;
}
});
let filteredCards = collectionData.cards.filter(card => card.survival_date == null && card.survival_mode_disabled == null && card.edition !== 9);
filteredCards.forEach(card => {
const splinter = cardDetailsMap[card.card_detail_id]?.splinter || "Unknown";
splinterCount[splinter] = (splinterCount[splinter] || 0) + 1;
});
filteredCards.sort((a, b) => {
const editionDiff = a.edition - b.edition;
if (editionDiff !== 0) return editionDiff;
const splinterA = cardDetailsMap[a.card_detail_id]?.splinter || "Unknown";
const splinterB = cardDetailsMap[b.card_detail_id]?.splinter || "Unknown";
if (splinterA !== splinterB) return splinterA.localeCompare(splinterB);
const nameA = cardDetailsMap[a.card_detail_id]?.name || "Unknown";
const nameB = cardDetailsMap[b.card_detail_id]?.name || "Unknown";
return nameA.localeCompare(nameB);
});
displayTables(filteredCards, cardDetailsMap, splinterCount);
} catch (error) {
alert("Error fetching data. Please try again later.");
console.error(error);
}
}
function displayTables(cards, cardDetailsMap, splinterCount) {
const summonerTableBody = document.querySelector('#summonerTable tbody');
const monsterTableBody = document.querySelector('#monsterTable tbody');
const colorCountTableBody = document.querySelector('#colorCountTable tbody');
summonerTableBody.innerHTML = "";
monsterTableBody.innerHTML = "";
colorCountTableBody.innerHTML = "";
for (const [splinter, count] of Object.entries(splinterCount)) {
const row = document.createElement('tr');
row.innerHTML = `
<td>${splinter}</td>
<td>${count}</td>
`;
colorCountTableBody.appendChild(row);
}
cards.forEach(card => {
const cardInfo = cardDetailsMap[card.card_detail_id] || { name: 'Unknown', type: 'Unknown', splinter: 'Unknown' };
const row = document.createElement('tr');
row.innerHTML = `
<td>${cardInfo.name}</td>
<td>${editionMapping[card.edition] || "Unknown"}</td>
<td>${cardInfo.splinter}</td>
<td>${card.level}</td>
`;
if (cardInfo.type === "Summoner") {
summonerTableBody.appendChild(row);
} else if (cardInfo.type === "Monster") {
monsterTableBody.appendChild(row);
}
});
}
</script>
</body>
</html>
obviously very basic and not very clean as I made a few adjustments to my initial idea as i was looking at the information. Even now its not correct as for some reason it doesnt include gold cards e.g - Tarsa missing on the list below although available if i go to battle.
either way, quite a fun little script and nearly gives me the information I need - if anyone knows what I, well chatGPT, is doing wrong let me know - I'm sure there will be an easier way to do this!
And, Yes, I know I have nowhere near enough cards to be competitive in Survival mode but we all start somewhere!
See you in the Arena